Home >> Blog >> 了解 java collection - 如何使用 java set
了解 java collection - 如何使用 java set
set 接口存在於java.util包中,並擴展了Collection 接口,它是一個無序的對象集合,其中不能存儲重複值。它是一個實現數學集的接口。該接口包含從 Collection 接口繼承的方法,並添加了限制重複元素插入的功能。有兩個接口擴展集合實現,即SortedSet和NavigableSet。
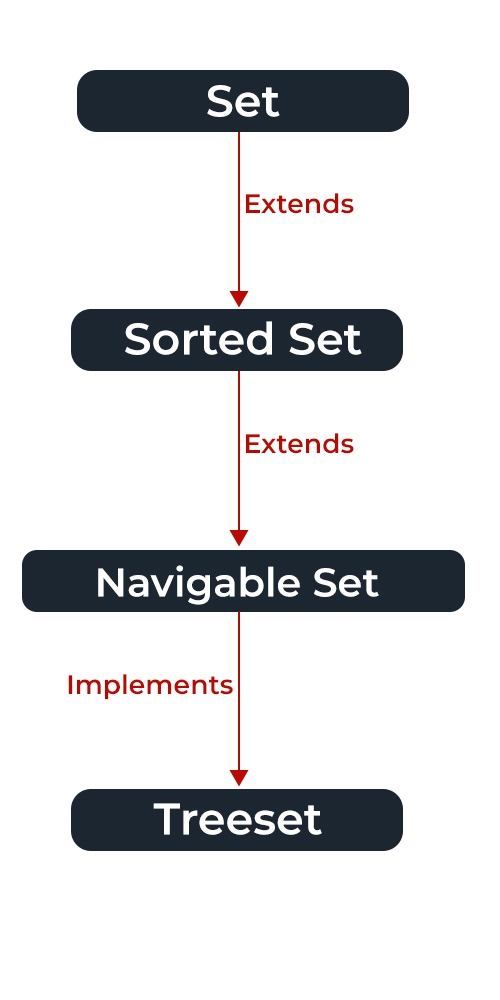
在上圖中,navigable set 擴展了 sorted set 接口。由於集合不保留插入順序,因此可導航集合接口提供了在集合中導航的實現。實現可導航集的類是 TreeSet,它是自平衡樹的實現。因此,這個界面為我們提供了一種在這棵樹中導航的方法。
聲明: Set 接口聲明為:
公共接口集擴展集合
創建集合對象
由於 Set 是一個interface,因此無法創建排版的對象。我們總是需要一個擴展這個列表的類來創建一個對象。而且,在 Java 1.5 中引入泛型之後,可以限制可以存儲在 Set 中的對像類型。這個類型安全的集合可以定義為:
// Obj是要存儲在Set中的對象的類型
Set
讓我們以下面的表格格式討論下面提供的 Set 接口中的方法,如下所示:
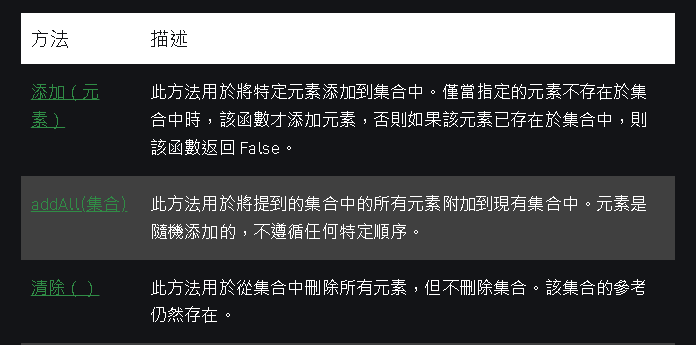
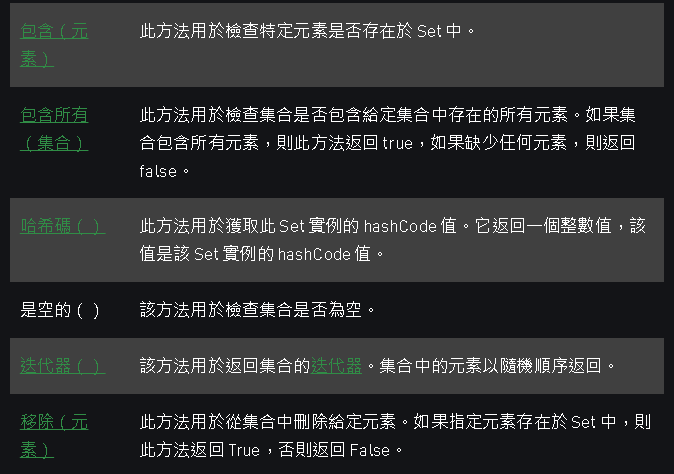
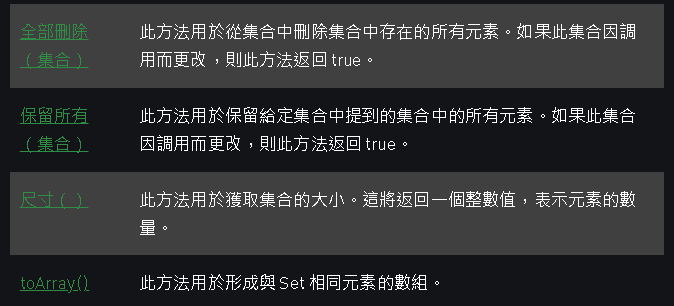
插圖:說明集界面的示例程序
// Java program Illustrating Set Interface
// Importing utility classes
import java.util.*;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Demonstrating Set using HashSet
// Declaring object of type String
Set< String> hash_Set = new HashSet< String>();
// Adding elements to the Set
// using add() method
hash_Set.add("Geeks");
hash_Set.add("For");
hash_Set.add("Geeks");
hash_Set.add("Example");
hash_Set.add("Set");
// Printing elements of HashSet object
System.out.println(hash_Set);
}
}
輸出
[設置,示例,極客,為]
設置界面上的操作
集合界面允許用戶對集合執行基本的數學運算。讓我們用兩個數組來理解這些基本操作。令 set1 = [1, 3, 2, 4, 8, 9, 0] 和 set2 = [1, 3, 7, 5, 4, 0, 7, 5]。那麼對集合可能的操作是:
1. 交集:此操作從給定的兩個集合中返回所有公共元素。對於上述兩組,交集將是:
交點 = [0, 1, 3, 4]
2.並集:此操作將一組中的所有元素與另一組相加。對於上述兩組,聯合將是:
聯合 = [0, 1, 2, 3, 4, 5, 7, 8, 9]
3.差異:此操作從另一組中刪除一組中存在的所有值。對於上述兩組,不同之處在於:
差異 = [2, 8, 9]
現在讓我們實現上面定義的以下操作,如下所示:
例子:
div hideAd=”auto”>
// Java Program Demonstrating Operations on the Set
// such as Union, Intersection and Difference operations
// Importing all utility classes
import java.util.*;
// Main class
public class SetExample {
// Main driver method
public static void main(String args[])
{
// Creating an object of Set class
// Declaring object of Integer type
Set< Integer> a = new HashSet< Integer>();
// Adding all elements to List
a.addAll(Arrays.asList(
new Integer[] { 1, 3, 2, 4, 8, 9, 0 }));
// Again declaring object of Set class
// with reference to HashSet
Set< Integer> b = new HashSet< Integer>();
b.addAll(Arrays.asList(
new Integer[] { 1, 3, 7, 5, 4, 0, 7, 5 }));
// To find union
Set< Integer> union = new HashSet< Integer>(a);
union.addAll(b);
System.out.print("Union of the two Set");
System.out.println(union);
// To find intersection
Set< Integer> intersection = new HashSet< Integer>(a);
intersection.retainAll(b);
System.out.print("Intersection of the two Set");
System.out.println(intersection);
// To find the symmetric difference
Set< Integer> difference = new HashSet< Integer>(a);
difference.removeAll(b);
System.out.print("Difference of the two Set");
System.out.println(difference);
}
}
輸出
兩個 Set[0, 1, 2, 3, 4, 5, 7, 8, 9] 的並集
兩個 Set[0, 1, 3, 4] 的交集
兩個 Set[2, 8, 9] 的區別
對 SortedSet 執行各種操作
Java 1.5引入泛型後,可以限制Set 中可以存儲的對像類型。由於 Set 是一個接口,它只能與實現該接口的類一起使用。HashSet 是廣泛使用的實現 Set 接口的類之一。現在,讓我們看看如何對 HashSet 執行一些常用的操作。我們將執行以下操作,如下所示:
- 添加元素
- 訪問元素
- 移除元素
- 迭代元素
- 遍歷 Set
現在讓我們分別討論這些操作,如下所示:
操作 1:添加元素
為了向 Set 添加元素,我們可以使用add() 方法。但是,插入順序不會保留在 Set 中。在內部,對於每個元素,都會生成一個散列,並根據生成的散列存儲值。這些值按升序進行比較和排序。我們需要注意,不允許重複元素,所有重複元素都將被忽略。而且,Set 接受 Null 值。
例子
// Java Program Demonstrating Working of Set by
// Adding elements using add() method
// Importing all utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating an object of Set and
// declaring object of type String
Set< String> hs = new HashSet< String>();
// Adding elements to above object
// using add() method
hs.add("B");
hs.add("B");
hs.add("C");
hs.add("A");
// Printing the elements inside the Set object
System.out.println(hs);
}
}
輸出
[A、B、C]
操作 2:訪問元素
添加元素後,如果我們希望訪問元素,可以使用內置方法,如contains()。
例子
// Java code to demonstrate Working of Set by
// Accessing the Elements of the Set object
// Importing all utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating an object of Set and
// declaring object of type String
Set< String> hs = new HashSet< String>();
// Elements are added using add() method
// Later onwards we will show accessing the same
// Custom input elements
hs.add("A");
hs.add("B");
hs.add("C");
hs.add("A");
// Print the Set object elements
System.out.println("Set is " + hs);
// Declaring a string
String check = "D";
// Check if the above string exists in
// the SortedSet or not
// using contains() method
System.out.println("Contains " + check + " "
+ hs.contains(check));
}
}
輸出
設置為 [A, B, C]
包含 D 錯誤
操作 3:刪除值
可以使用remove() 方法從 Set 中刪除這些值。
例子
// Java Program Demonstrating Working of Set by
// Removing Element/s from the Set
// Importing all utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Declaring object of Set of type String
Set< S tring> hs = new HashSet< String>();
// Elements are added
// using add() method
// Custom input elements
hs.add("A");
hs.add("B");
hs.add("C");
hs.add("B");
hs.add("D");
hs.add("E");
// Printing initial Set elements
System.out.println("Initial HashSet " + hs);
// Removing custom element
// using remove() method
hs.remove("B");
// Printing Set elements after removing an element
// and printing updated Set elements
System.out.println("After removing element " + hs);
}
}
初始哈希集 [A, B, C, D, E]
刪除元素 [A, C, D, E] 後
操作 4:遍歷集合
有多種方法可以遍歷 Set。最著名的一種是使用增強的 for 循環。
例子
// Java Program to Demonstrate Working of Set by
// Iterating through the Elements
// Importing utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating object of Set and declaring String type
Set< String> hs = new HashSet< String>();
// Adding elements to Set
// using add() method
// Custom input elements
hs.add("A");
hs.add("B");
hs.add("C");
hs.add("B");
hs.add("D");
hs.add("E");
// Iterating through the Set
// via for-each loop
for (String value : hs)
// Printing all the values inside the object
System.out.print(value + ", ");
System.out.println();
}
}
輸出
甲、乙、丙、丁、乙、
Java Collections中實現Set接口的類從下圖可以很容易地感知到,列舉如下:
- 哈希集
- 枚舉集
- 鏈接哈希集
- 樹集
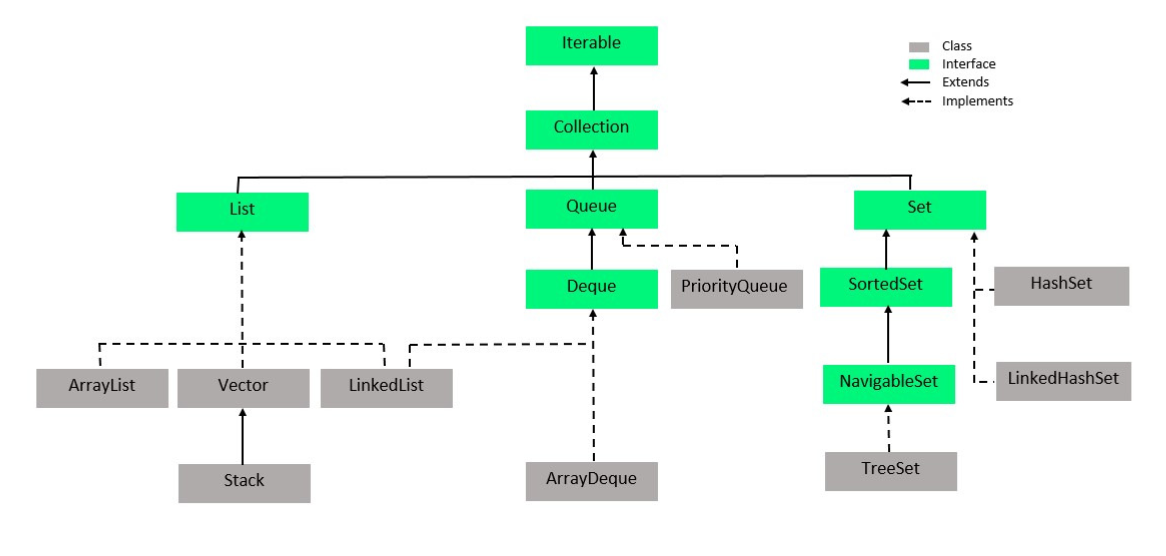
第 1 類:哈希集
在集合框架中實現的 HashSet 類是哈希表數據結構的固有實現。我們插入到 HashSet 中的對像不保證以相同的順序插入。對像是根據它們的哈希碼插入的。此類還允許插入 NULL 元素。讓我們看看如何使用這個類創建一個集合對象。
例子
// Java program Demonstrating Creation of Set object
// Using the Hashset class
// Importing utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating object of Set of type String
Set< String> h = new HashSet< String>();
// Adding elements into the HashSet
// using add() method
// Custom input elements
h.add("India");
h.add("Australia");
h.add("South Africa");
// Adding the duplicate element
h.add("India");
// Displaying the HashSet
System.out.println(h);
// Removing items from HashSet
// using remove() method
h.remove("Australia");
System.out.println("Set after removing "
+ "Australia:" + h);
// Iterating over hash set items
System.out.println("Iterating over set:");
// Iterating through iterators
Iterator< String> i = h.iterator();
// It holds true till there is a single element
// remaining in the object
while (i.hasNext())
System.out.println(i.next());
}
}
輸出
[南非、澳大利亞、印度]
移除澳大利亞後設置:[南非、印度]
迭代集合:
南非
印度
第 2 類:枚舉集
在集合框架中實現的 EnumSet 類是用於枚舉類型的 Set 接口的專門實現之一。它是一個高性能的集合實現,比 HashSet 快得多。枚舉集中的所有元素都必須來自一個枚舉類型,該枚舉類型是在顯式或隱式創建集合時指定的。讓我們看看如何使用這個類創建一個集合對象。
例子
// Java program to demonstrate the
// creation of the set object
// using the EnumSet class
import java.util.*;
enum Gfg { CODE, LEARN, CONTRIBUTE, QUIZ, MCQ }
;
public class GFG {
public static void main(String[] args)
{
// Creating a set
Set< Gfg> set1;
// Adding the elements
set1 = EnumSet.of(Gfg.QUIZ, Gfg.CONTRIBUTE,
Gfg.LEARN, Gfg.CODE);
System.out.println("Set 1: " + set1);
}
}
第三類: LinkedHashSet
在集合框架中實現的 LinkedHashSet 類是HashSet 的有序版本,它維護一個跨所有元素的雙向鍊錶。當需要維護迭代順序時,使用此類。當遍歷 HashSet 時,順序是不可預測的,而 LinkedHashSet 讓我們按照元素插入的順序遍曆元素。讓我們看看如何使用這個類創建一個集合對象。
例子
// Java program to demonstrate the
// creation of Set object using
// the LinkedHashset class
import java.util.*;
class GFG {
public static void main(String[] args)
{
Set< String> lh = new LinkedHashSet< String>();
// Adding elements into the LinkedHashSet
// using add()
lh.add("India");
lh.add("Australia");
lh.add("South Africa");
// Adding the duplicate
// element
lh.add("India");
// Displaying the LinkedHashSet
System.out.println(lh);
// Removing items from LinkedHashSet
// using remove()
lh.remove("Australia");
System.out.println("Set after removing "
+ "Australia:" + lh);
// Iterating over linked hash set items
System.out.println("Iterating over set:");
Iterator
while (i.hasNext())
System.out.println(i.next());
}
}
輸出
[印度、澳大利亞、南非]
移除澳大利亞後設置:[印度、南非]
迭代集合:
印度
南非
第 4 類:樹集
在集合框架中實現的TreeSet 類和SortedSet 接口和 SortedSet 的實現擴展了 Set 接口。它的行為類似於一個簡單的集合,不同之處在於它以排序格式存儲元素。TreeSet 使用樹數據結構進行存儲。對象按排序的升序存儲。但是我們可以使用 TreeSet.descendingIterator() 方法以降序迭代。讓我們看看如何使用這個類創建一個集合對象。
例子
// Java Program Demonstrating Creation of Set object
// Using the TreeSet class
// Importing utility classes
import java.util.*;
// Main class
class GFG {
// Main driver method
public static void main( String[] args)
{
// Creating a Set object and declaring it of String
// type
// with reference to TreeSet
Set< String> ts = new TreeSet< String>();
// Adding elements into the TreeSet
// using add()
ts.add("India");
ts.add("Australia");
ts.add("South Africa");
// Adding the duplicate
// element
ts.add("India");
// Displaying the TreeSet
System.out.println(ts);
// Removing items from TreeSet
// using remove()
ts.remove("Australia");
System.out.println("Set after removing "
+ "Australia:" + ts);
// Iterating over Tree set items
System.out.println("Iterating over set:");
Iterator< String> i = ts.iterator();
while (i.hasNext())
System.out.println(i.next());
}
}
輸出
[澳大利亞、印度、南非]
移除澳大利亞後設置:[印度、南非]
迭代集合:
印度
南非T